mirror of
https://salsa.debian.org/gnuk-team/gnuk/gnuk.git
synced 2024-09-19 18:30:15 +00:00
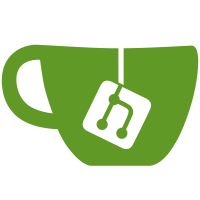
ECDH curves exercised are OpenPGP recommended set: ANSIx9p{256,384,521}r1 and BRAINPOOLp{256,384,512}r1, plus X25519 and ED25519. Signature is only tested (for now ?) with ED25519 as other signature schemes are (usually) non-deterministic and require implementing the signature verification algorithm rather than just testing for equality with a test vector. Signed-off-by: Vincent Pelletier <plr.vincent@gmail.com>
71 lines
3.5 KiB
Python
71 lines
3.5 KiB
Python
"""
|
|
card_test_brainpoolp512r1.py - test brainpoolp512r1 support
|
|
|
|
Copyright (C) 2021 Vincent Pelletier <plr.vincent@gmail.com>
|
|
|
|
This file is a part of Gnuk, a GnuPG USB Token implementation.
|
|
|
|
Gnuk is free software: you can redistribute it and/or modify it
|
|
under the terms of the GNU General Public License as published by
|
|
the Free Software Foundation, either version 3 of the License, or
|
|
(at your option) any later version.
|
|
|
|
Gnuk is distributed in the hope that it will be useful, but WITHOUT
|
|
ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
|
|
or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public
|
|
License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
"""
|
|
|
|
from func_pso_auth import assert_ec_pso
|
|
from card_const import *
|
|
|
|
class Test_Card_BrainpoolP512R1(object):
|
|
def test_ECDH_reference_vectors(self, card):
|
|
assert card.verify(3, FACTORY_PASSPHRASE_PW3)
|
|
assert card.verify(2, FACTORY_PASSPHRASE_PW1)
|
|
# https://tools.ietf.org/html/rfc7027#appendix-A.3
|
|
assert_ec_pso(
|
|
card=card,
|
|
key_index=1,
|
|
key_attributes=KEY_ATTRIBUTES_ECDH_BRAINPOOLP512R1,
|
|
key_attribute_caption='ECDH brainpoolp512r1',
|
|
private_key=(
|
|
b'\x16\x30\x2F\xF0\xDB\xBB\x5A\x8D\x73\x3D\xAB\x71\x41\xC1\xB4\x5A'
|
|
b'\xCB\xC8\x71\x59\x39\x67\x7F\x6A\x56\x85\x0A\x38\xBD\x87\xBD\x59'
|
|
b'\xB0\x9E\x80\x27\x96\x09\xFF\x33\x3E\xB9\xD4\xC0\x61\x23\x1F\xB2'
|
|
b'\x6F\x92\xEE\xB0\x49\x82\xA5\xF1\xD1\x76\x4C\xAD\x57\x66\x54\x22'
|
|
),
|
|
expected_public_key=(
|
|
b'\x04'
|
|
b'\x0A\x42\x05\x17\xE4\x06\xAA\xC0\xAC\xDC\xE9\x0F\xCD\x71\x48\x77'
|
|
b'\x18\xD3\xB9\x53\xEF\xD7\xFB\xEC\x5F\x7F\x27\xE2\x8C\x61\x49\x99'
|
|
b'\x93\x97\xE9\x1E\x02\x9E\x06\x45\x7D\xB2\xD3\xE6\x40\x66\x8B\x39'
|
|
b'\x2C\x2A\x7E\x73\x7A\x7F\x0B\xF0\x44\x36\xD1\x16\x40\xFD\x09\xFD'
|
|
b'\x72\xE6\x88\x2E\x8D\xB2\x8A\xAD\x36\x23\x7C\xD2\x5D\x58\x0D\xB2'
|
|
b'\x37\x83\x96\x1C\x8D\xC5\x2D\xFA\x2E\xC1\x38\xAD\x47\x2A\x0F\xCE'
|
|
b'\xF3\x88\x7C\xF6\x2B\x62\x3B\x2A\x87\xDE\x5C\x58\x83\x01\xEA\x3E'
|
|
b'\x5F\xC2\x69\xB3\x73\xB6\x07\x24\xF5\xE8\x2A\x6A\xD1\x47\xFD\xE7'
|
|
),
|
|
pso_input=(
|
|
b'\xa6\x81\x88\x7f\x49\x81\x84\x86\x81\x81'
|
|
b'\x04'
|
|
b'\x9D\x45\xF6\x6D\xE5\xD6\x7E\x2E\x6D\xB6\xE9\x3A\x59\xCE\x0B\xB4'
|
|
b'\x81\x06\x09\x7F\xF7\x8A\x08\x1D\xE7\x81\xCD\xB3\x1F\xCE\x8C\xCB'
|
|
b'\xAA\xEA\x8D\xD4\x32\x0C\x41\x19\xF1\xE9\xCD\x43\x7A\x2E\xAB\x37'
|
|
b'\x31\xFA\x96\x68\xAB\x26\x8D\x87\x1D\xED\xA5\x5A\x54\x73\x19\x9F'
|
|
b'\x2F\xDC\x31\x30\x95\xBC\xDD\x5F\xB3\xA9\x16\x36\xF0\x7A\x95\x9C'
|
|
b'\x8E\x86\xB5\x63\x6A\x1E\x93\x0E\x83\x96\x04\x9C\xB4\x81\x96\x1D'
|
|
b'\x36\x5C\xC1\x14\x53\xA0\x6C\x71\x98\x35\x47\x5B\x12\xCB\x52\xFC'
|
|
b'\x3C\x38\x3B\xCE\x35\xE2\x7E\xF1\x94\x51\x2B\x71\x87\x62\x85\xFA'
|
|
),
|
|
expected_pso_output=(
|
|
b'\xA7\x92\x70\x98\x65\x5F\x1F\x99\x76\xFA\x50\xA9\xD5\x66\x86\x5D'
|
|
b'\xC5\x30\x33\x18\x46\x38\x1C\x87\x25\x6B\xAF\x32\x26\x24\x4B\x76'
|
|
b'\xD3\x64\x03\xC0\x24\xD7\xBB\xF0\xAA\x08\x03\xEA\xFF\x40\x5D\x3D'
|
|
b'\x24\xF1\x1A\x9B\x5C\x0B\xEF\x67\x9F\xE1\x45\x4B\x21\xC4\xCD\x1F'
|
|
),
|
|
)
|